I finally got access to the Unity 3.0 preview build last weekend, and was able to spend a couple of hours with it. My goal for the session was to add a lightmap to my A Tack game. Here’s how it went.
Installation
Always rename your old Unity install folder(s) before running a new Unity installer. (This goes for all Unity upgrades, not just the Unity 3 preview.) This lets you return to old versions. For a disposable beta version this is obviously useful, but I’ve sometimes found it handy to open a project in an old version for comparison.
Obviously you should also make a backup of projects before letting Unity convert them into a new format, which Unity 3 does.
Converting the Right Project
There is a pitfall I ran into even though I had made a backup of the project I wanted to convert to Unity 3. I tried to open it, but then ran into the registration screen. Once I’d gotten past that, Unity opened and converted the last project I’d worked on instead of the one I’d just picked to open.
I imagine that it was the registering screen that caused this problem. After registering be sure to read the dialog carefully and note which project Unity is about to convert.
MonoDevelop
I have been eagerly looking forward to both the debugging and code completion features, so the first thing I did was set up Unity to use MonoDevelop. The steps to do this are:
- Create a MonoDevelop solution file.
- Open that solution in MonoDevelop, build it, then run it.
- (optional) In Unity’s preferences, set MonoDevelop to be the default script editor, so that opening a script doesn’t bring up Unitron.
The manual has a section on this; I also found the following video demonstration (courtesy of BergZergArcade.com) helpful:
Autocompletion and C# syntax highlighting in MonoDevelop worked well. These will save me many trips to Unity and Mono references. (I didn’t try debugging with breakpoints yet.)
Bug: unrecognized method FileInfo.OpenText
The first project I opened (unintentionally) was Pawns. I was able to build it in MonoDevelop, but the Unity IDE refused to run it, claiming that one of my scripts was using an unknown function. System.IO.FileInfo.OpenText is a perfectly legitimate method though, and I’ve been using it for months with Unity 2.6 and Unity for iPhone. (MonoDevelop’s autocomplete also thought it was legit.)
This was a showstopper unfortunately, so I closed Pawns for the time being and converted the project I’d originally intended: A Tack.
Conversion Then Repair
Perhaps its because I’m editing projects that were originally created with Unity 1.x and have gone through many edits and conversions since, but almost any time I convert to a newer Unity format I lose some asset assignments. This time was no exception so I spent a little while re-assigning models and scripts to objects that had lost them. This was my first exposure to the new texture picker, which makes selecting the right texture much easier:
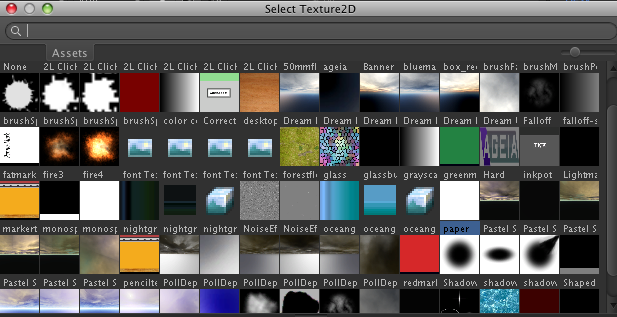
Scalable, searchable texture picker
A Tack reads and writes pixels from some of its textures. This is a setting on the texture that defaults to off. so I had to go through my brush and paper textures to enable read/write on each. (Unity gave a helpful error message for this so there was no mystery.)
Speaking of texture settings, there are a few new import settings for textures. The settings now include support for normal maps and lightmaps, and towards the bottom there are settings that can be overridden when building for different platforms (I’m licensed for Mac/PC and iPhone, so next to the Default there are two additional icons.)
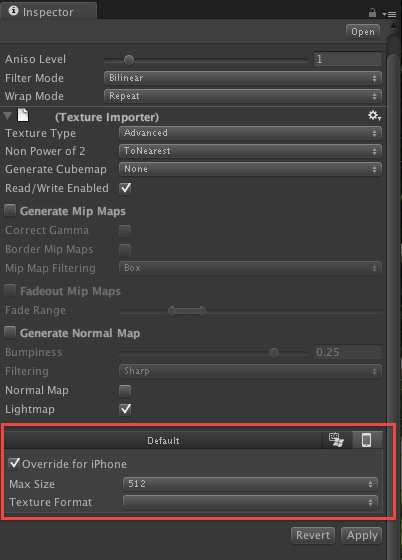
Unity 3 Preview's texture import settings
Finally it was time to try out the feature I’d been looking forward to: lightmapping.
Lightmapping
The main reason the spotlight exists in my scene is to make that nice-looking circle of light on the desk; a lightmap for the desk will let me get rid of the spotlight, saving on lighting calculations.
The steps I followed to lightmap the desk were:
- Toggle the lightmapping checkbox on the texture I wanted to lightmap (in this case, the desk.)
- Select the desk (a plane) in the scene Hierarchy view, then click on the menu Tools->Lightmapping.
- Select the static checkbox, then click bake.
The lightmapping calculations churned for about a minute. I then turned off the spotlight in my scene, but the lighted circle on the desk remained. Success! So simple.
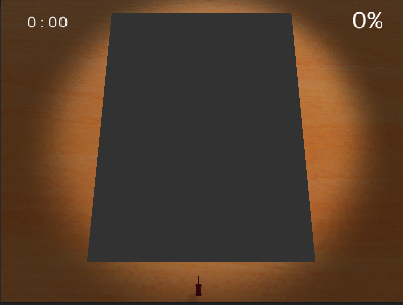
Spotlight facing the desk was replaced by a lightmap
I finally called it a night when the paper object stopped reflecting any light. (Haven’t solved this one yet; it seemed to start when I tried to lightmap that texture, and nothing I’ve tried since has fixed the problem.)
Conclusions
This was a preview release, and probably not even the most current beta. I ran into pretty much the kinds of bugs and conversion issues that I expected. Some were minor; only one was a definite showstopper. So overall my impressions are positive.
I’m thrilled about the lightmapper and the use of MonoDevelop for coding and debugging. Plus there are dozens of other useful features that I haven’t played with yet. I’m feeling the same sense of excitement and discovery that I felt the first time I tried out Unity, way back in 2005.